The plugin comes with a javascript API to easily manipulate the form, with the API you can do stuff like setting the value of a field, changing the options of a dropdown, listening to change events of a given field, etc.
There are two ways of accessing the API:
- Through an external script file
- Throw the Javascript tab of your form.
Accessing the API through an external javascript file
You can access the API through the variable “LoadedForms”, this variable is an array that has the API of each of the forms loaded into the page. To find the API of a given form you can search by id with a code like this
LoadedForms.find(x=>x.FormId==97)
The code below returns the API of the form with id 97.
Accessing the API through the javascript tab.
An easier way to access the API is to add your code in the javascript tab of your form
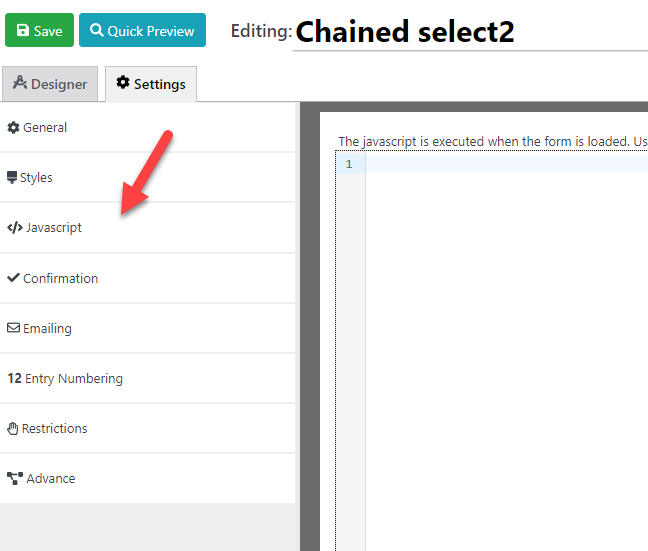
Under the javascript tab, you can access the API using the variable “this”
Getting a field with the API
In order to manipulate a field you first need to access it, you can get any field through the API using the “GetFieldById” method of the API, for example:
var myField=this.GetFieldById(15)
This will get the field with id 15. You can find the id of any field in the form designer
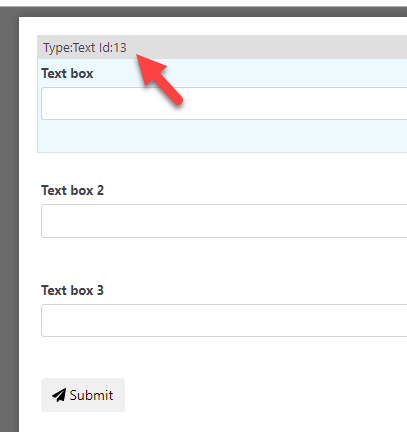
Manipulating a field
Depending on the field you are accessing you will find various methods that you can use to get or set information. For example, a text field has the method GetText() and SetText(text) methods to get and set its value respectively:
LoadedForms[0].GetFieldById(15).SetText("My test")
The code above will set the value of the text field with id of 15 to “My test”
Debugging your code
Depending on what you want to do it might be tricky to find issues in it. A nice way of debugging it is to add the keyword debugger; to your code. This line, when executing the form with the developer tools open (In chrome you can open the developer tools by pressing F12) will pause the execution and let you inspect what the browser is doing in each line.
Example:
This code will pause the browser just before its execution
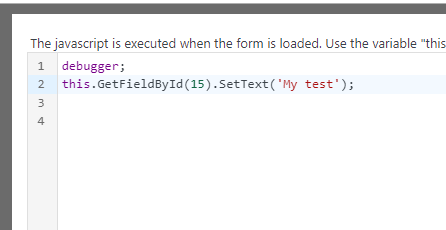
When paused I can execute the code line by line to be sure it is working as expected and I can even check what is inside each variable
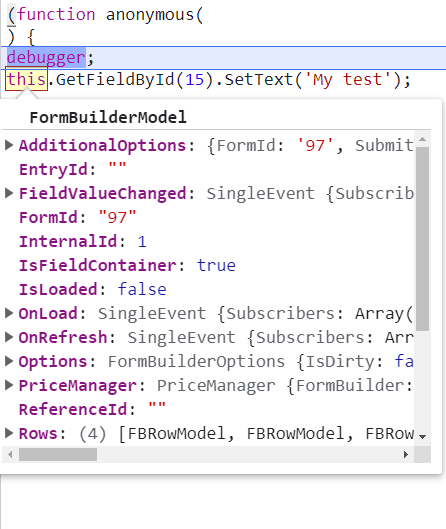
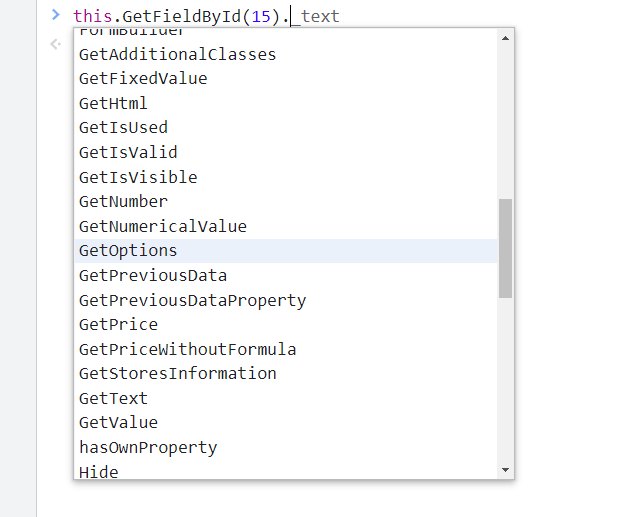
FEB
2023